## What is graphQL
- Query language for your API
- Server side runtime
- Type system
- Not tied to any specific storage/technology
## How does a graphql request looks like?
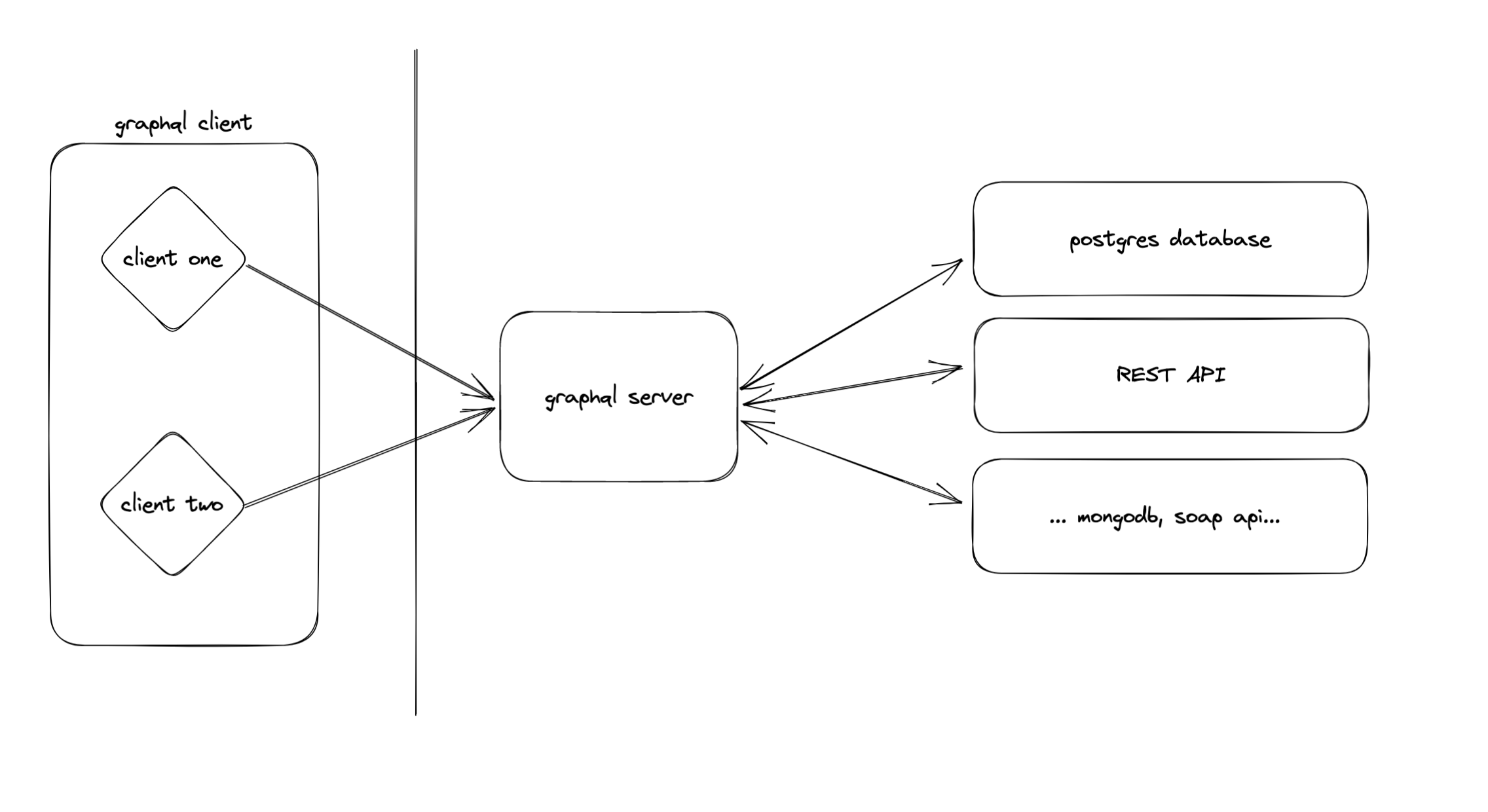
## Querying data from a client
- Same shape query and results
- You can query fields directly or have nested fields
- You can also have a list returning from a field

## Arguments
- Query level or field level
- The server will receive it and can then filter the data
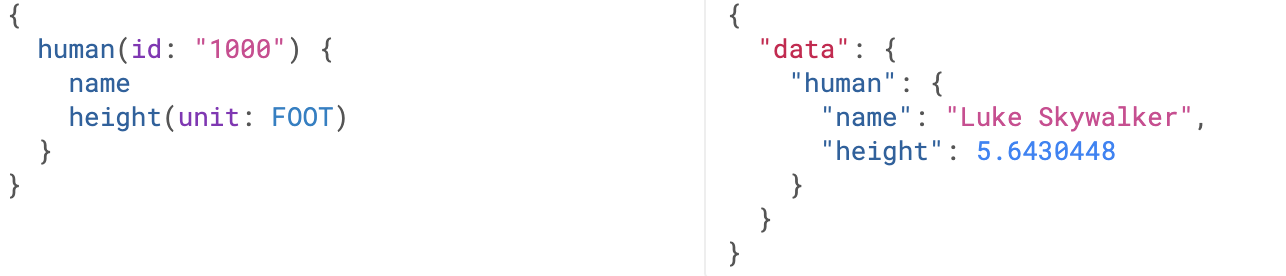
## Fragments
- Reusable unit
- You can construct a set of fields and include them
- You need to specify the type (
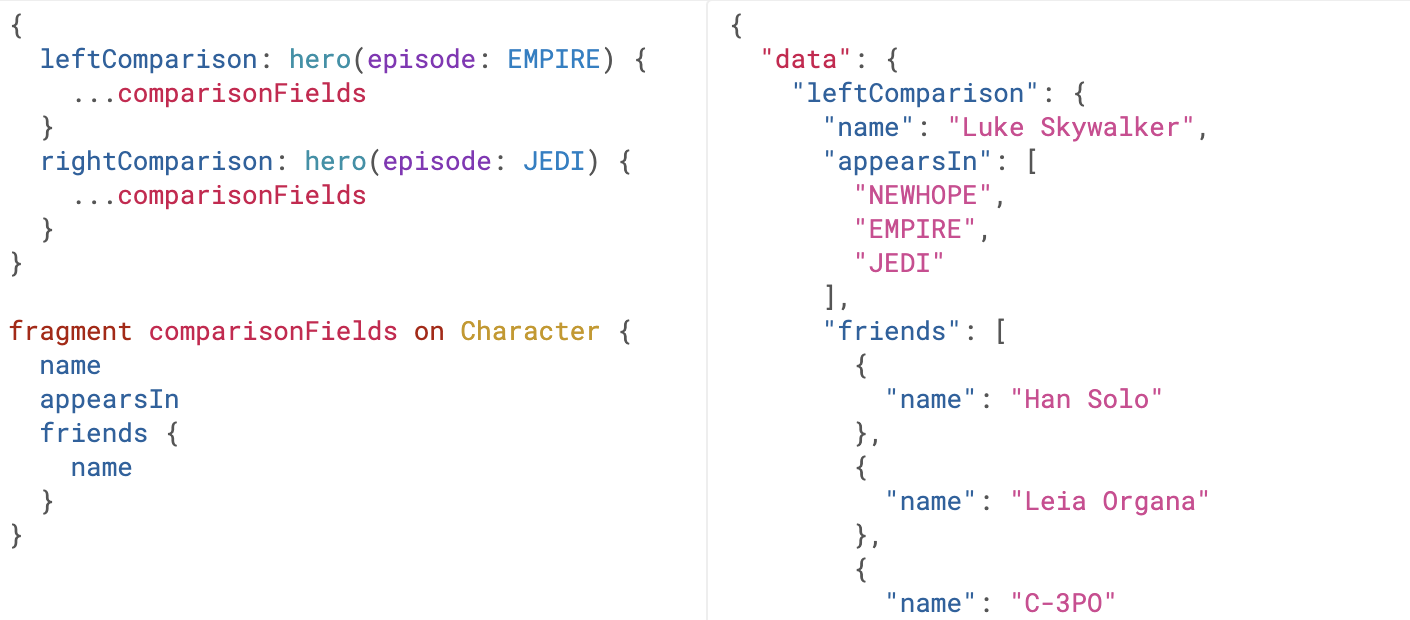
## Operation name
- Operation types: query, mutations and subscriptions
- Meaninful operation name for debugging
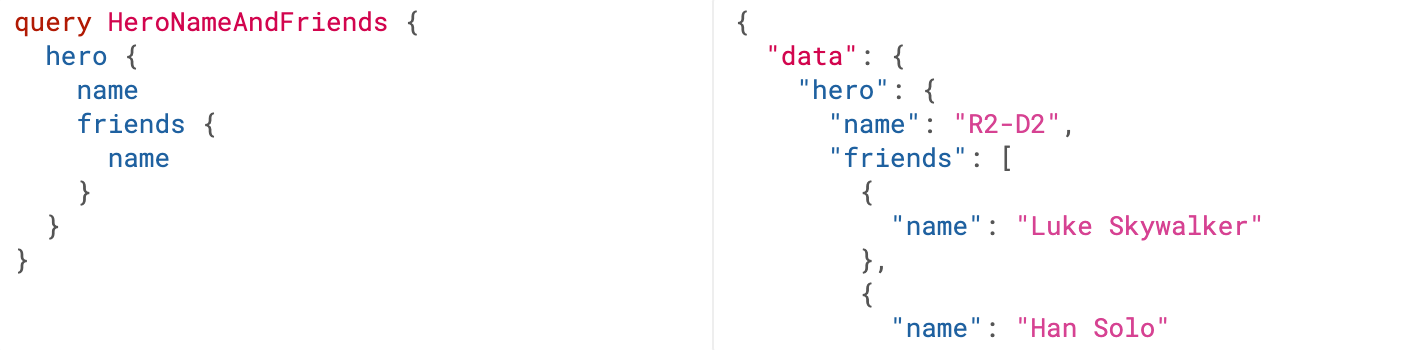
## Variables
- Not great to manipulate the graphql string directly
- Way to pass dynamic values into the query
- Replace value with `$variableName`, declare it in the query, pass variable in the transport-specific way
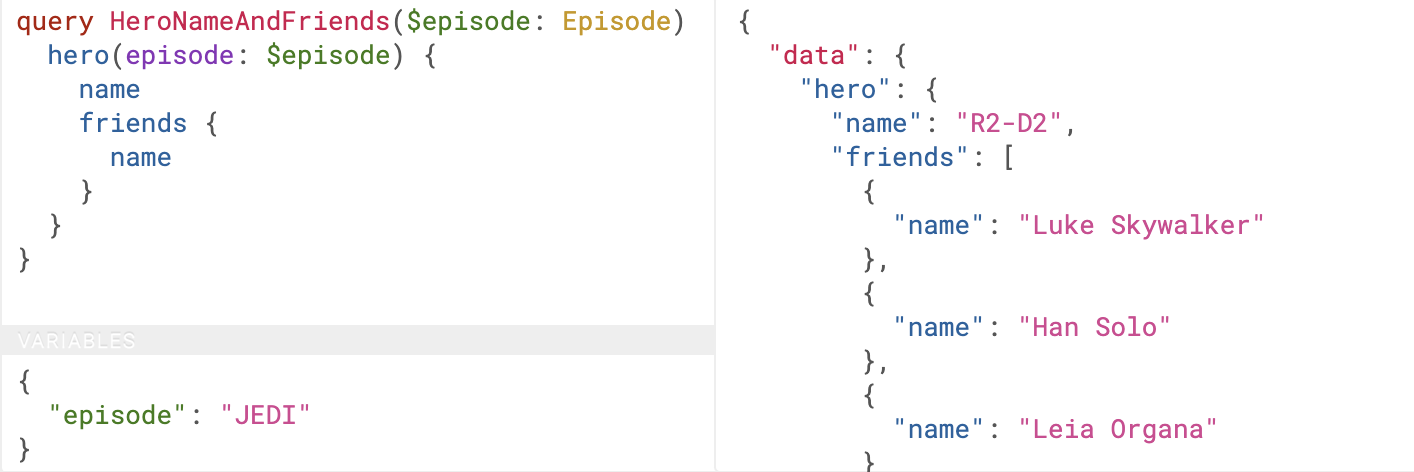
## Simple directives
- We might want a way to dinamically change a query
- Can be attached to a field or fragment
- By spec servers need to implement at least `@include` and `@skip`
- You can implement your own custom directives
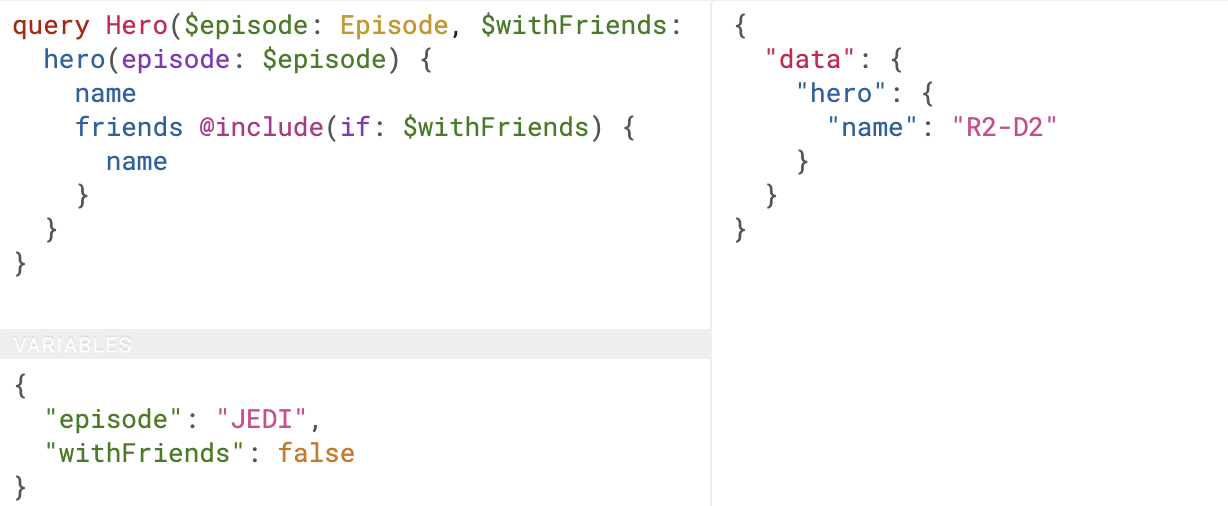
## Mutations
- You could modify data with a query
- Convention similar to REST GET/POST/PATCH so you don't modify data by mistake
- Mutation returns an object
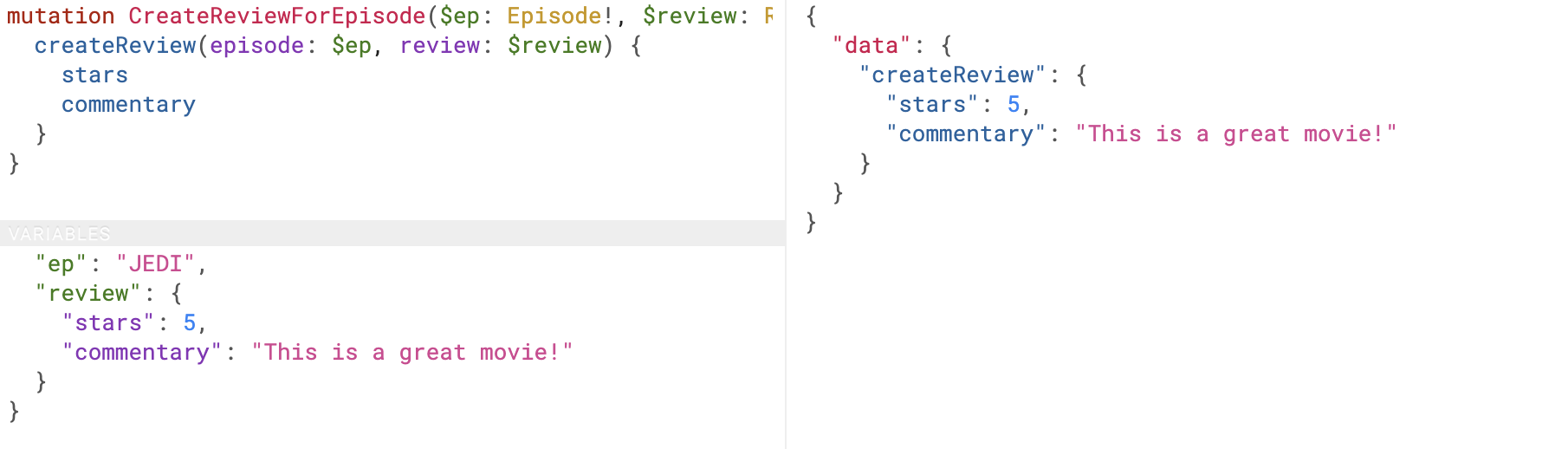
## Graphql server
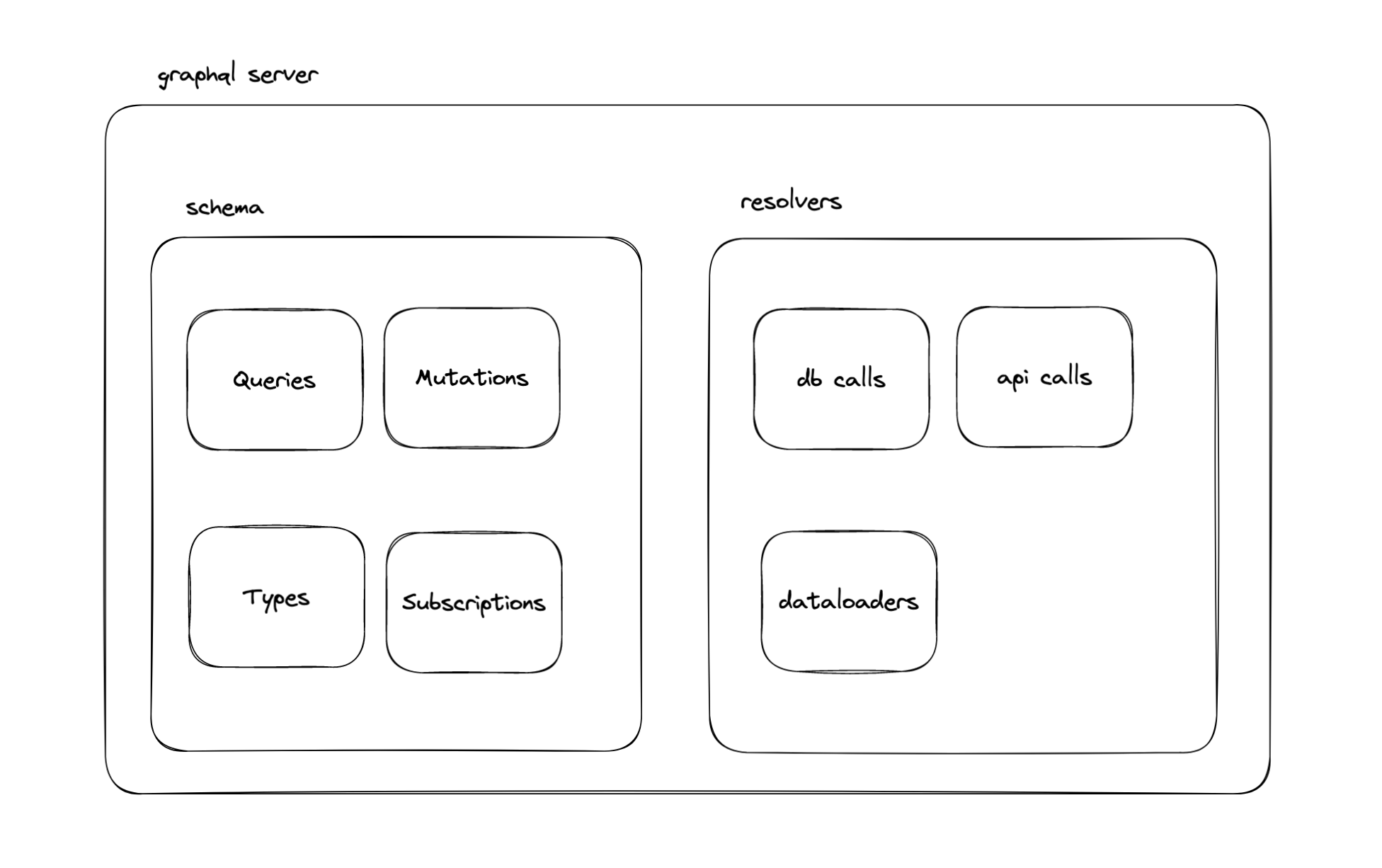
## Query and mutation
- Special types within a schema, query and mutation
- They define entry points for query and mutations


## Types
- These are defined on the server side on the schema
- A type can have multiple fields
- `!` makes the field mandatory
- Default scalar types of `Int`, `Float`, `String`, `Boolean`, `ID` and `Scalar`

## Enums
- Limits the values to a specific list
- Get sent over the wire as a string
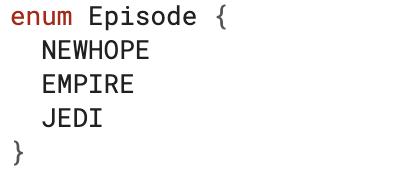
## Interfaces
- Abstract types that need to be implemented
- Ensure all the implemented types share some fields
- All the fields need to be re-defined in the concrete types
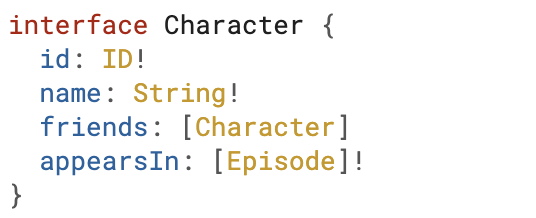
## Union types
- Define a type to be one of multiple concrete types

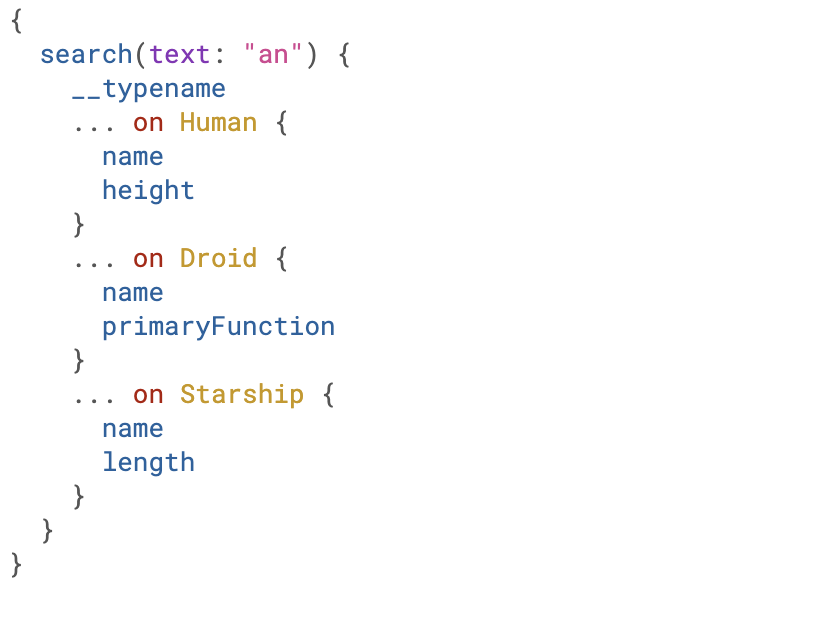
## Input types
- Types that can be an argument to a query or field
- Helpful for mutation when you're creating a new object
- Just like a regular type but the fields cannot have arguments
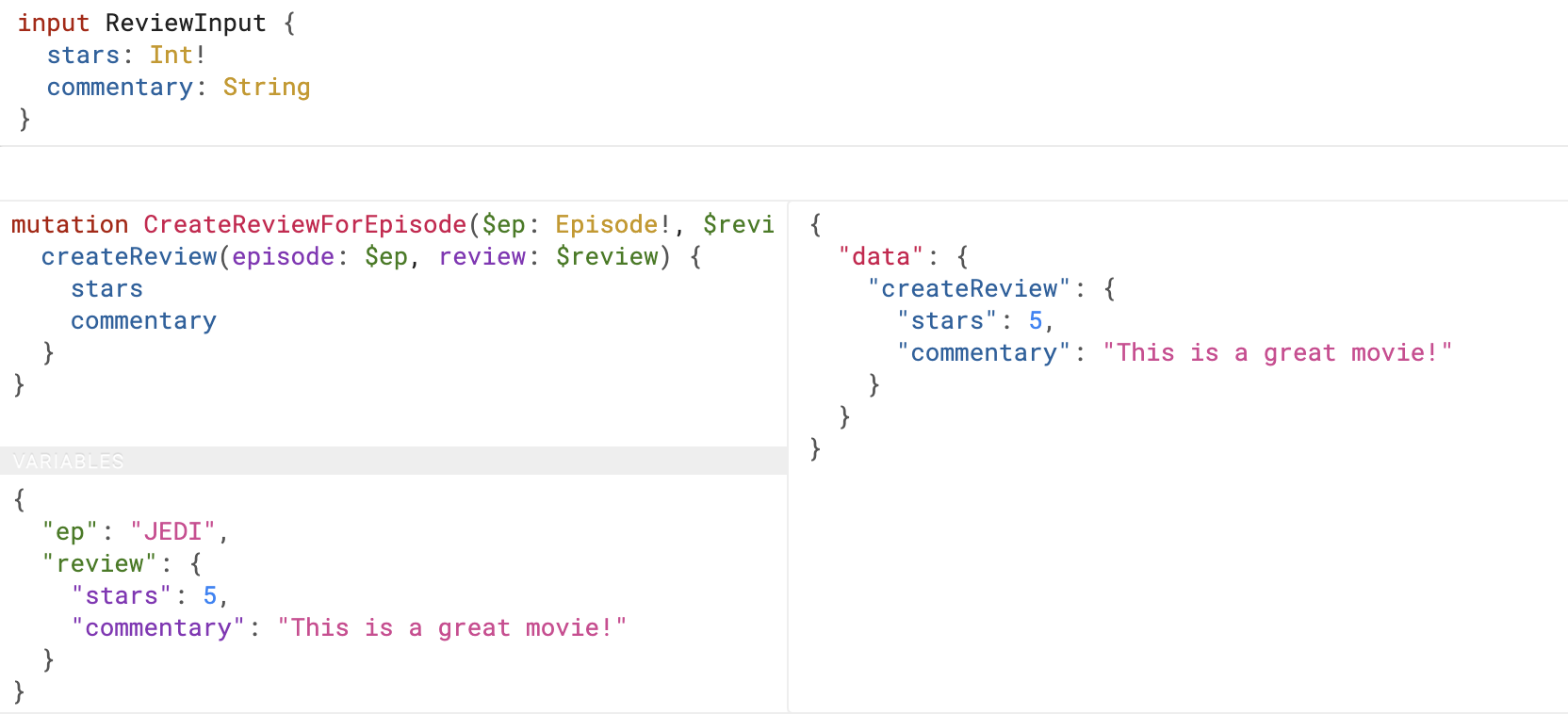
## Resolvers
- Function that get called to resolve the type with the actual data
- Contains parent object, arguments, context and info
- Can resolve on the query type, or on the type itself
- Can be asyncronous
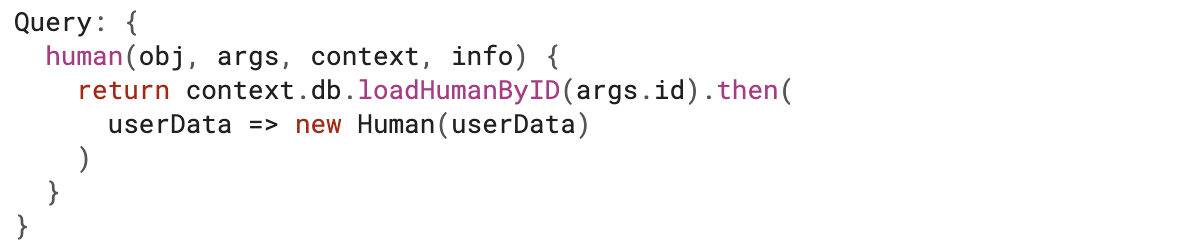
## Dataloaders
- Resolvers gets called once per each field
- N+1 problem when fetching on type resolvers
- Dataloader: gather all the requests and execute them on the next tick
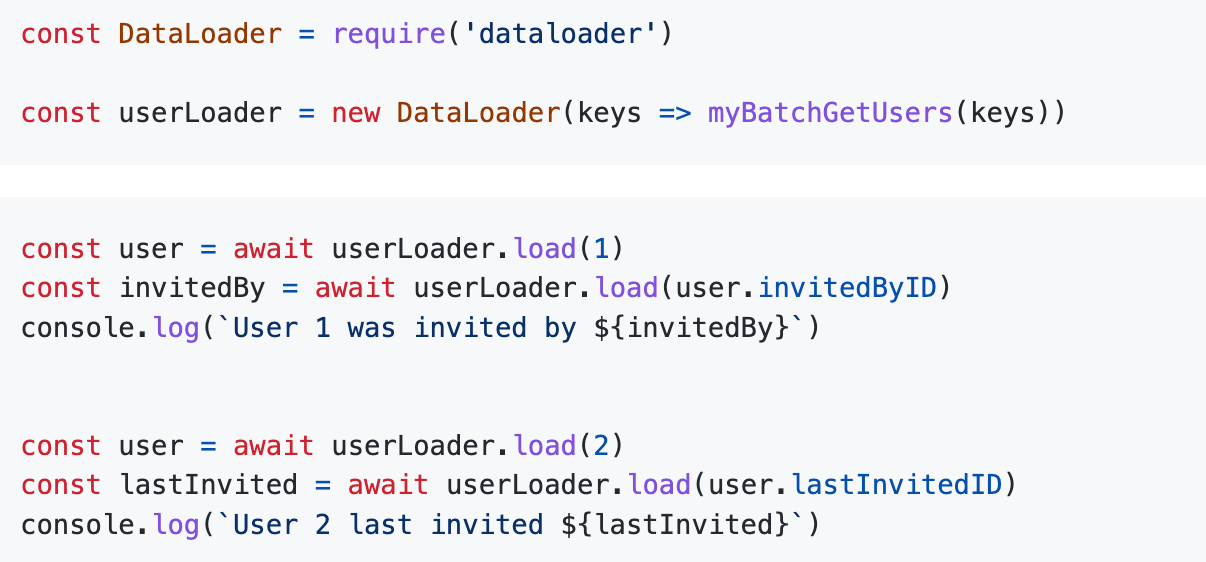
## Where it shines
- Rapid prototyping
- Good if you have backend teams and frontend teams (contract)
- Clients can get caching for free
- Great for devices with very small bandwiths
## Where it does not shine
- Defining the structure of the requests in the client can lead to issues
- It doesn't have the best performance (especially node/apollo)
- It adds a layer of complexity
- Caching in the network is harder as requests are post
- All the requests return 200 (even for errors)
## GraphQL Federation: WHY?
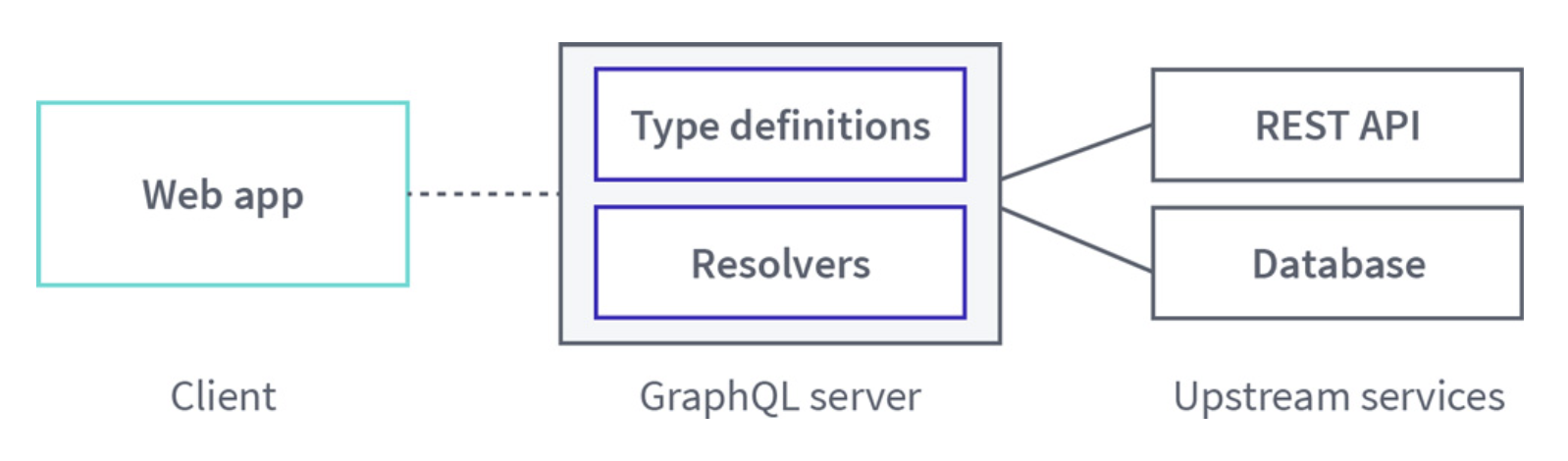
- This is where all applications start
- Perfect for simple application
- Great to have a unified view of the data
## THE MONOLITH
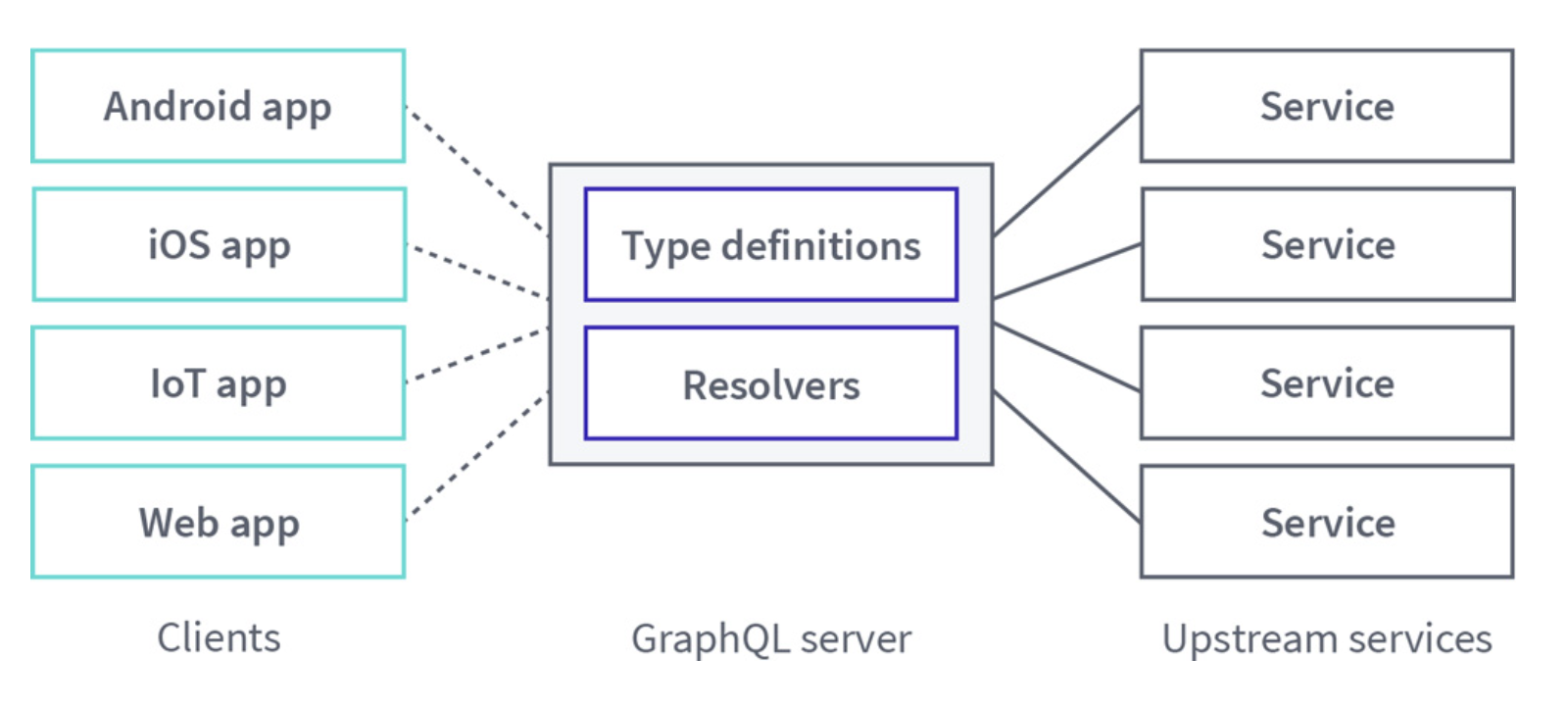
- As the application grows, it starts to tangle more and more
- Multiple teams working on it, with risk of type duplications, and moving slower
## BFF
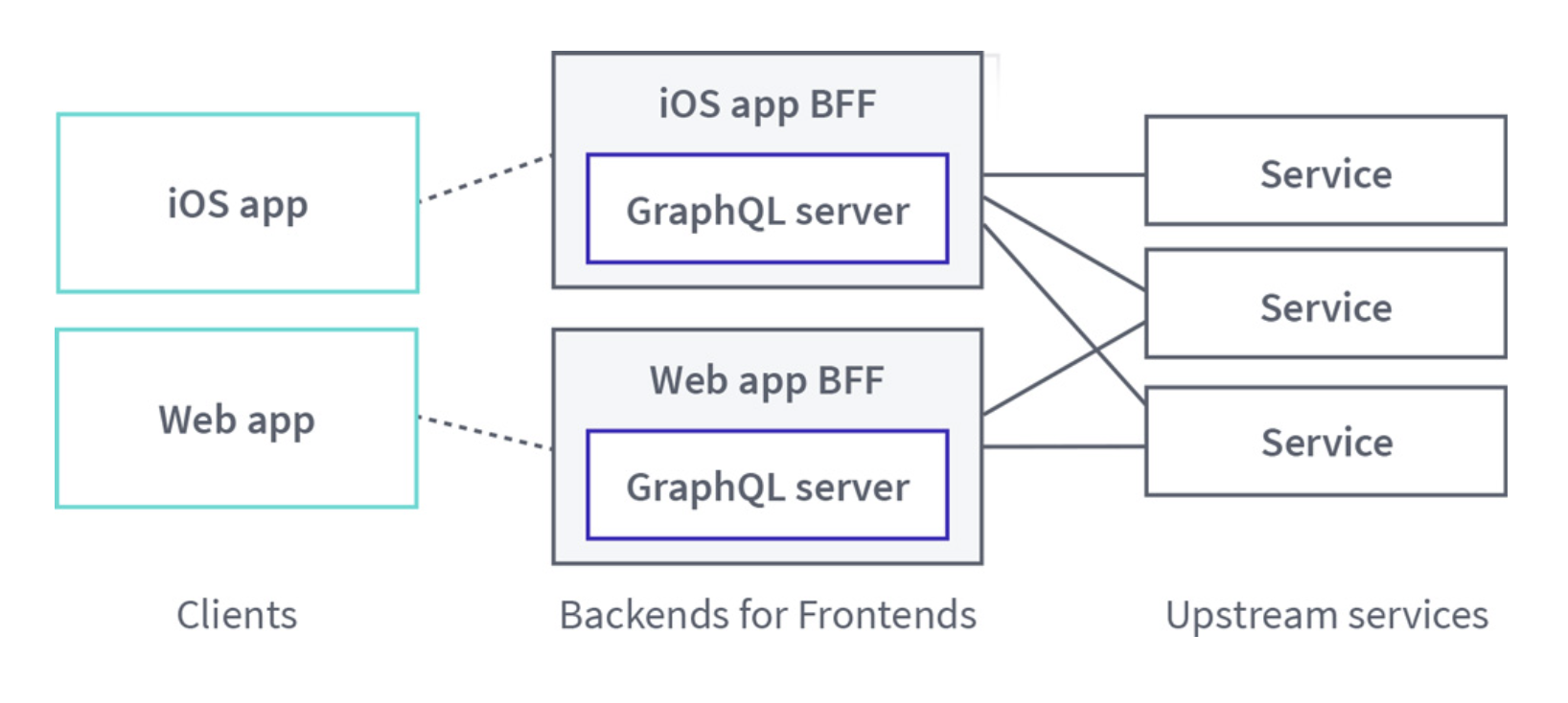
- Good developer experience, domain separation
- Duplicate effort, missing an unified view of the data
## Apollo Federation: Concepts
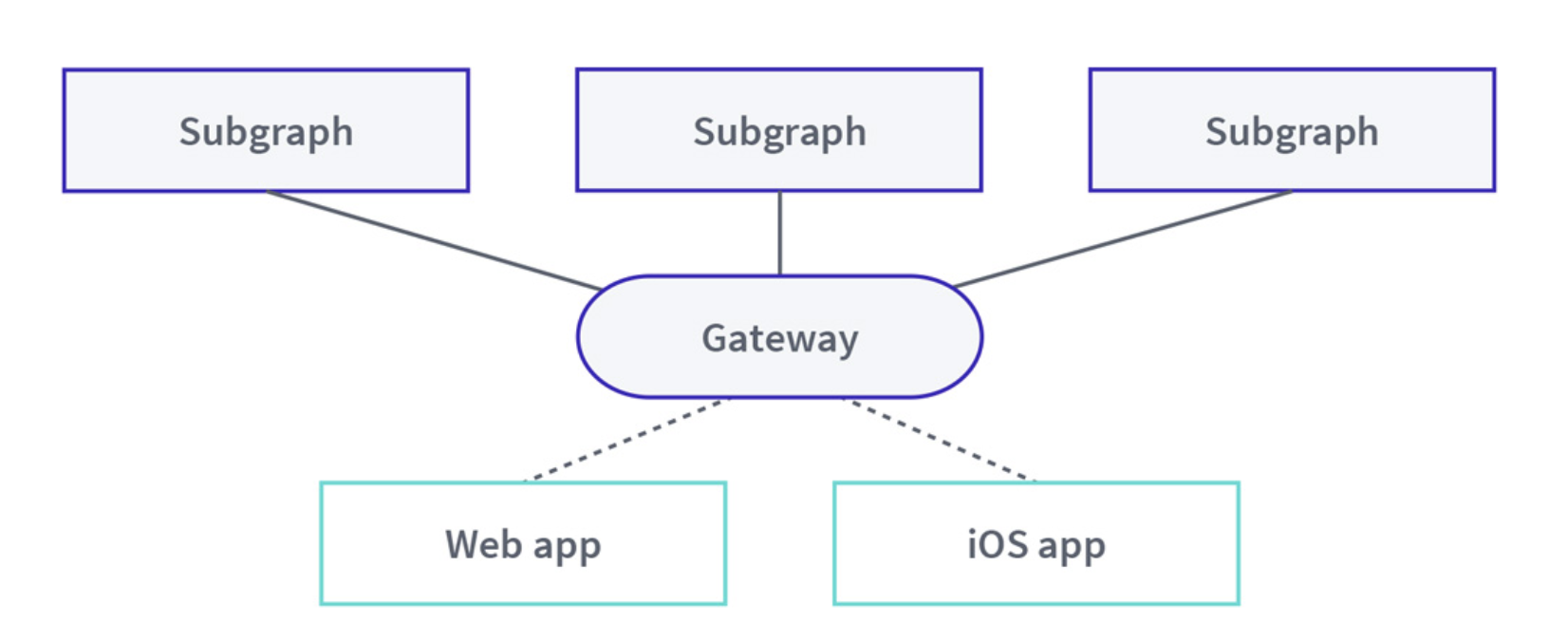
- The gateway is the entry point of each request and coordinates all the subgraphs
- What is a subgraph? It's an instance of @apollo/subgraph. For example, In SE we got disco, se and assessment subgraphs
## Apollo Federation: Concepts
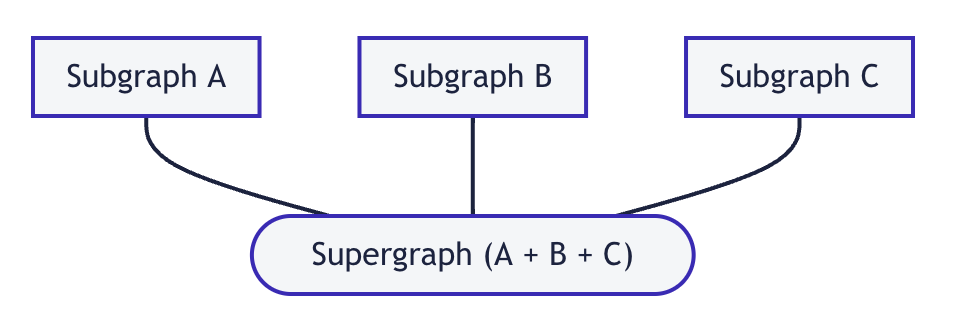
- In a federated architecture multiple subgraphs are federated into a single "supergraph" on the gateway
- Easy to merge different types, but what about extending?
## Apollo Federation: Entities
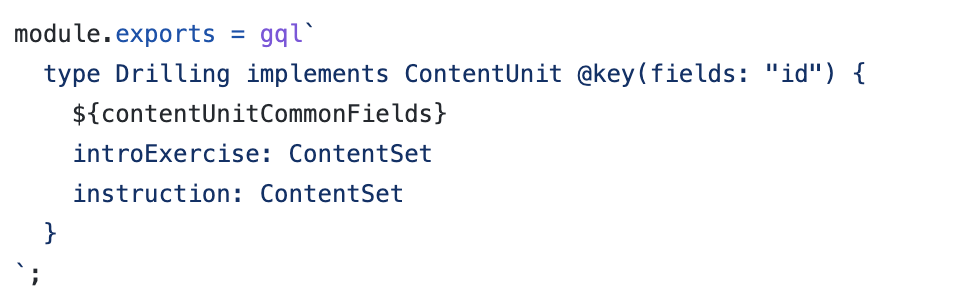
- An entity is an object type that you define in one subgraph and you extend and reference in other subgraphs
- In apollo, you can define an entity by using the `@key` directive
## Apollo Federation: Entities
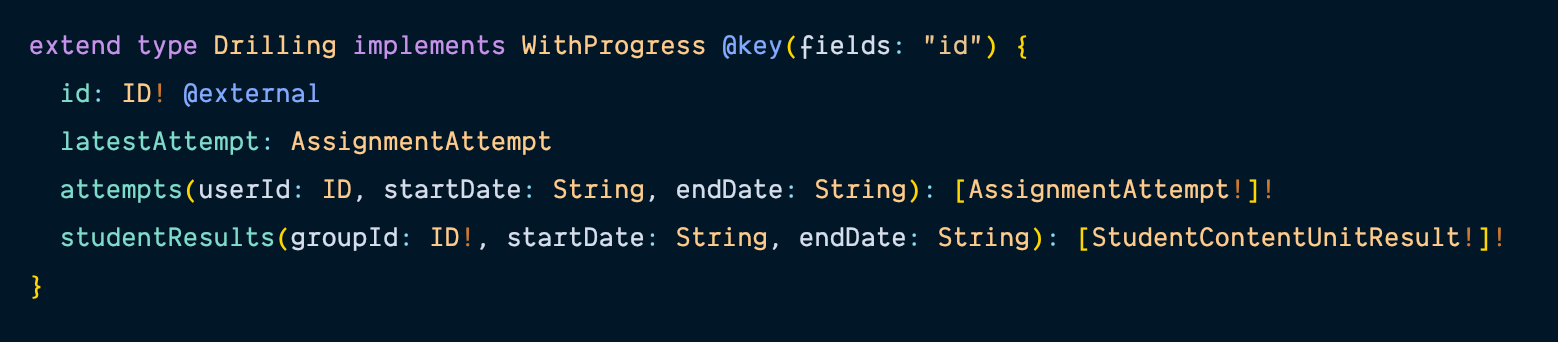
- SE subgraph extends the type from Disco
- SE needs also resolvers for the fields it provides
## Apollo Federation: Entities
- Merged type in federation
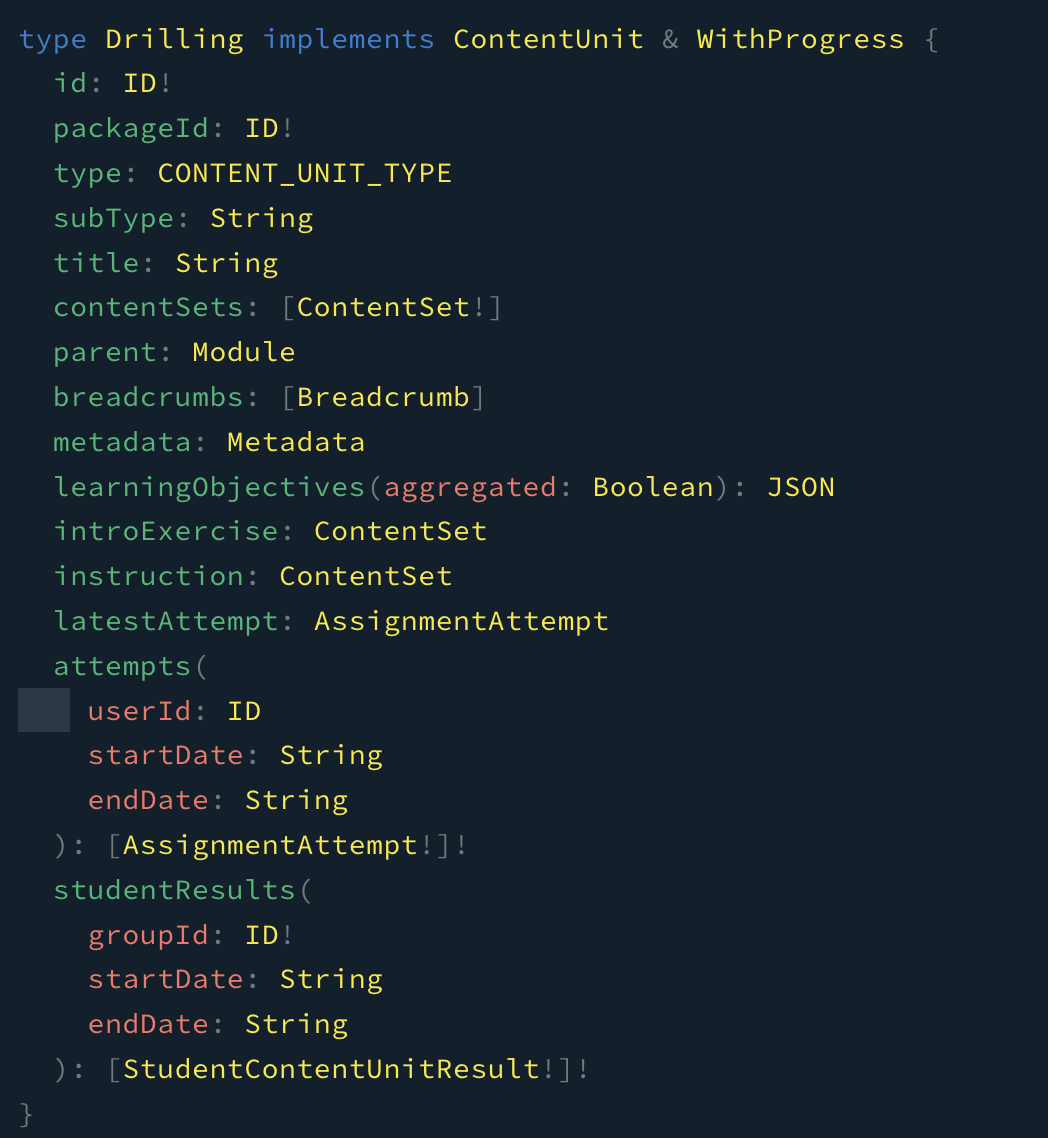
## Apollo Federation: Concepts
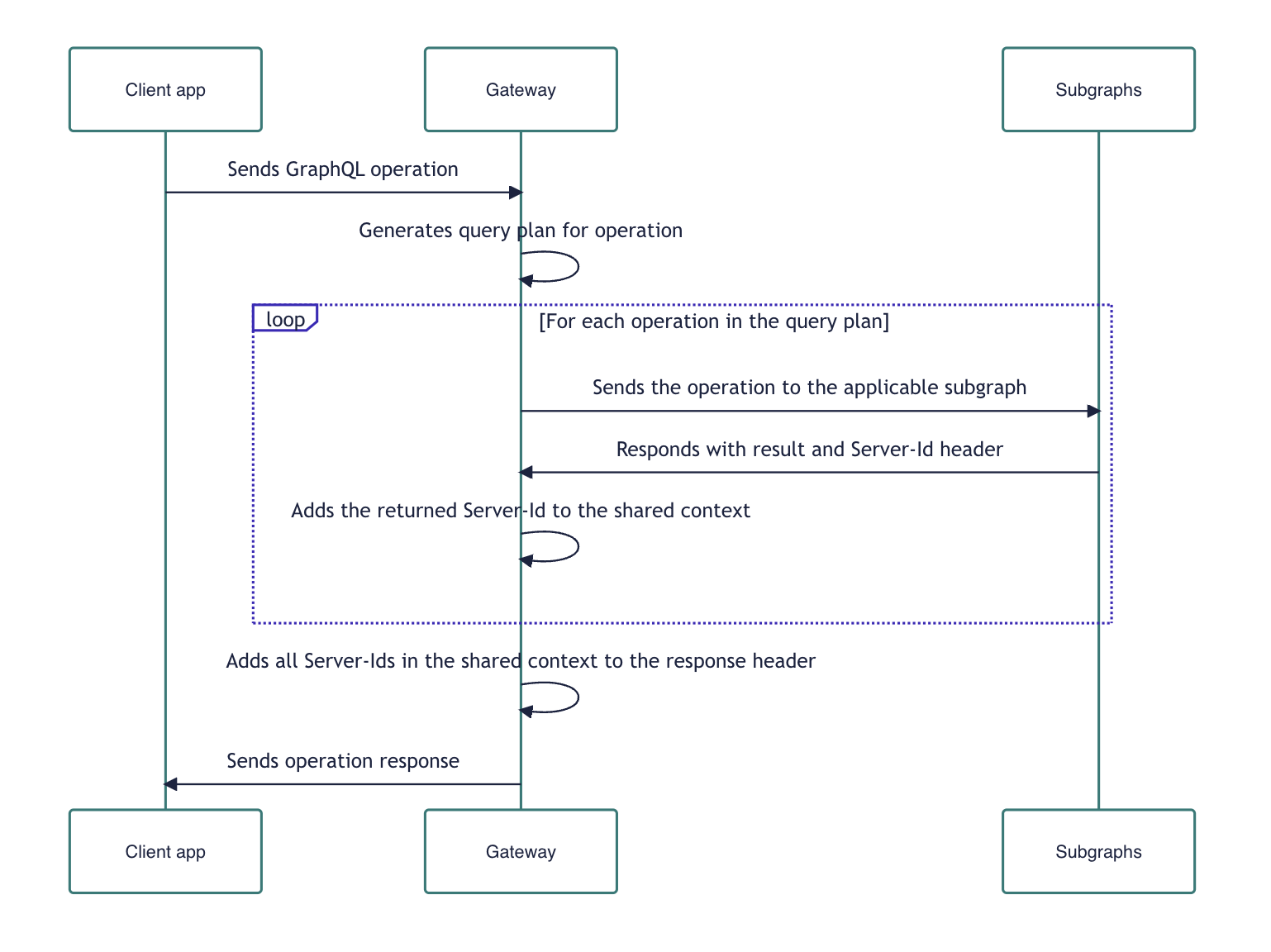
## INNER WORKINGS
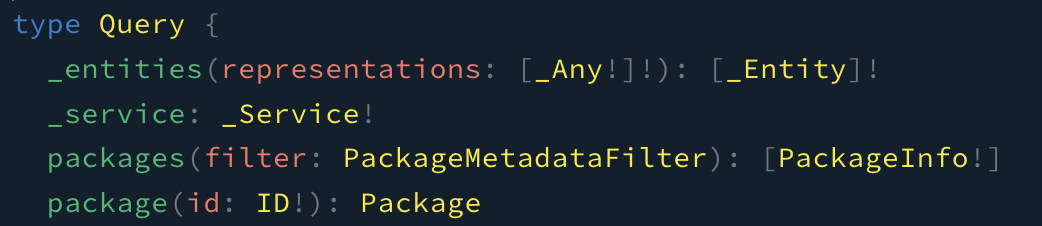
- Transforming the server to a subgraph, it will provide a _service and a _entities query
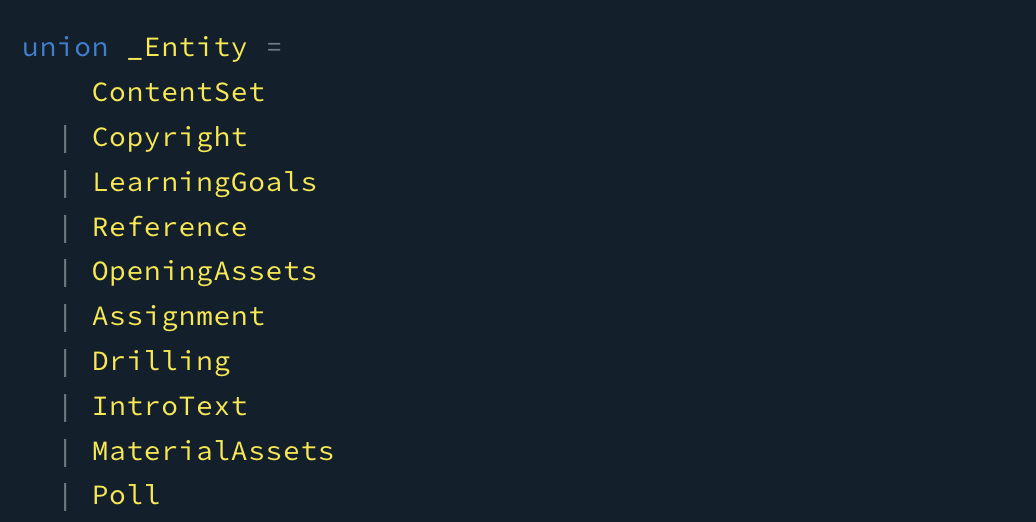
## How data is queried
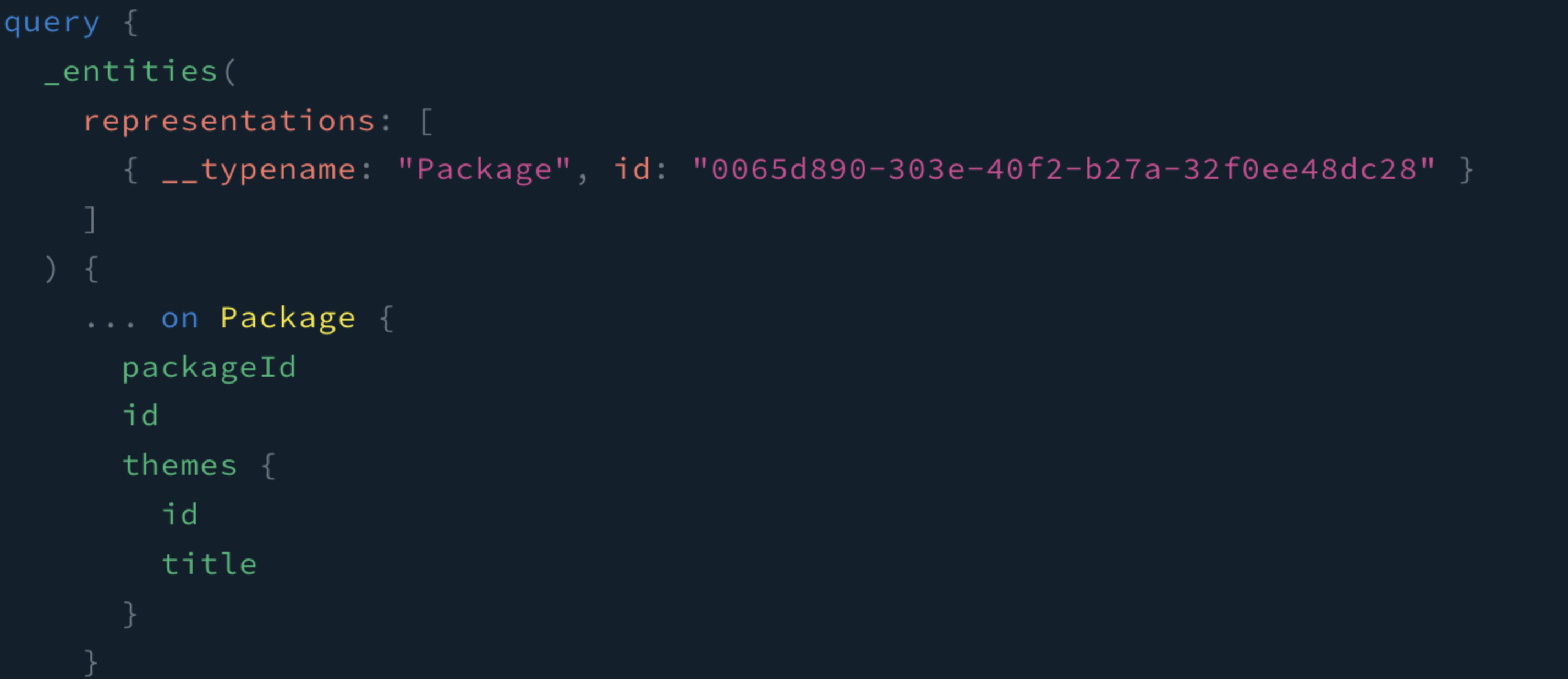
- We query for any type specifying the required keys and the typename
- Use the `...on` sytax to query the fields of the specific type
## So are we using apollo federation?
- Only on the subgraphs, the gateway is using @graphql-tools stitching
- Reasons: smaller bundle size & less bloated, more powerful, runs smooths on workers
- Compatible with apollo federation (not to be confused with the "old" stitching)
## How does graphql-tools stitching works?
- Remote schemas that threats remote endpoints as if they were local executable schemas
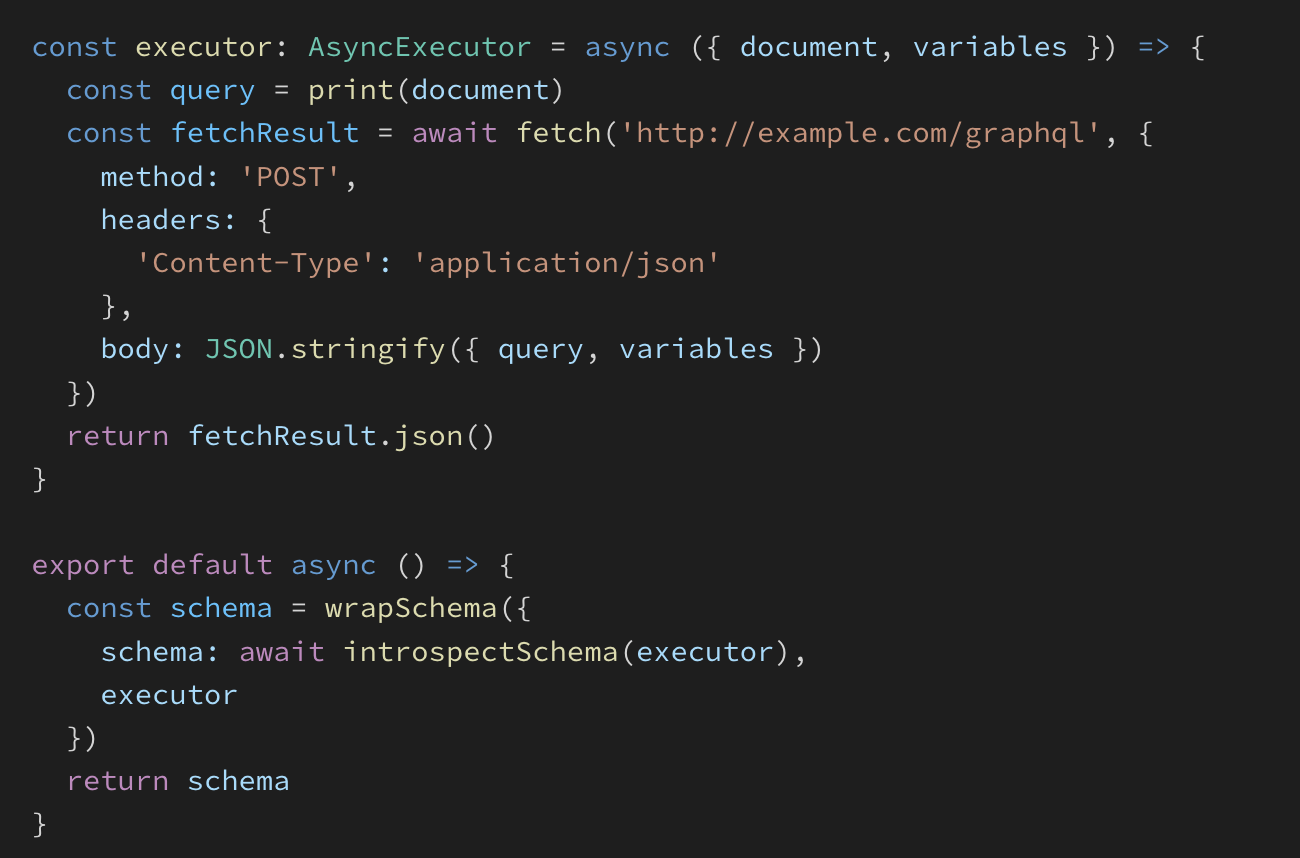
## How does graphql-tools stitching works?
- Stitching schemas together will create a single schema like on apollo federation
- Some minor conversion needed for the directives
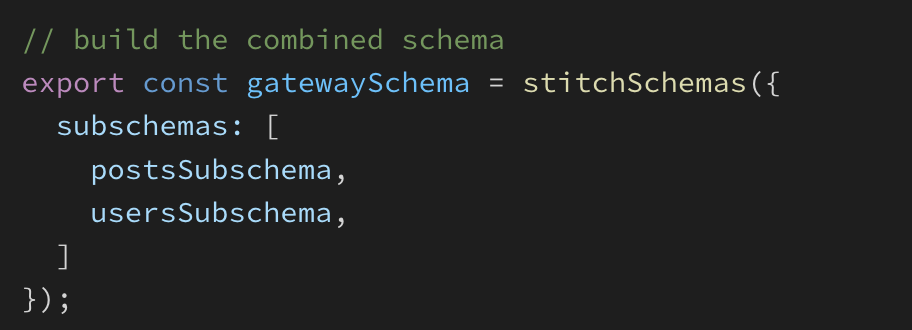
## Full stitching example
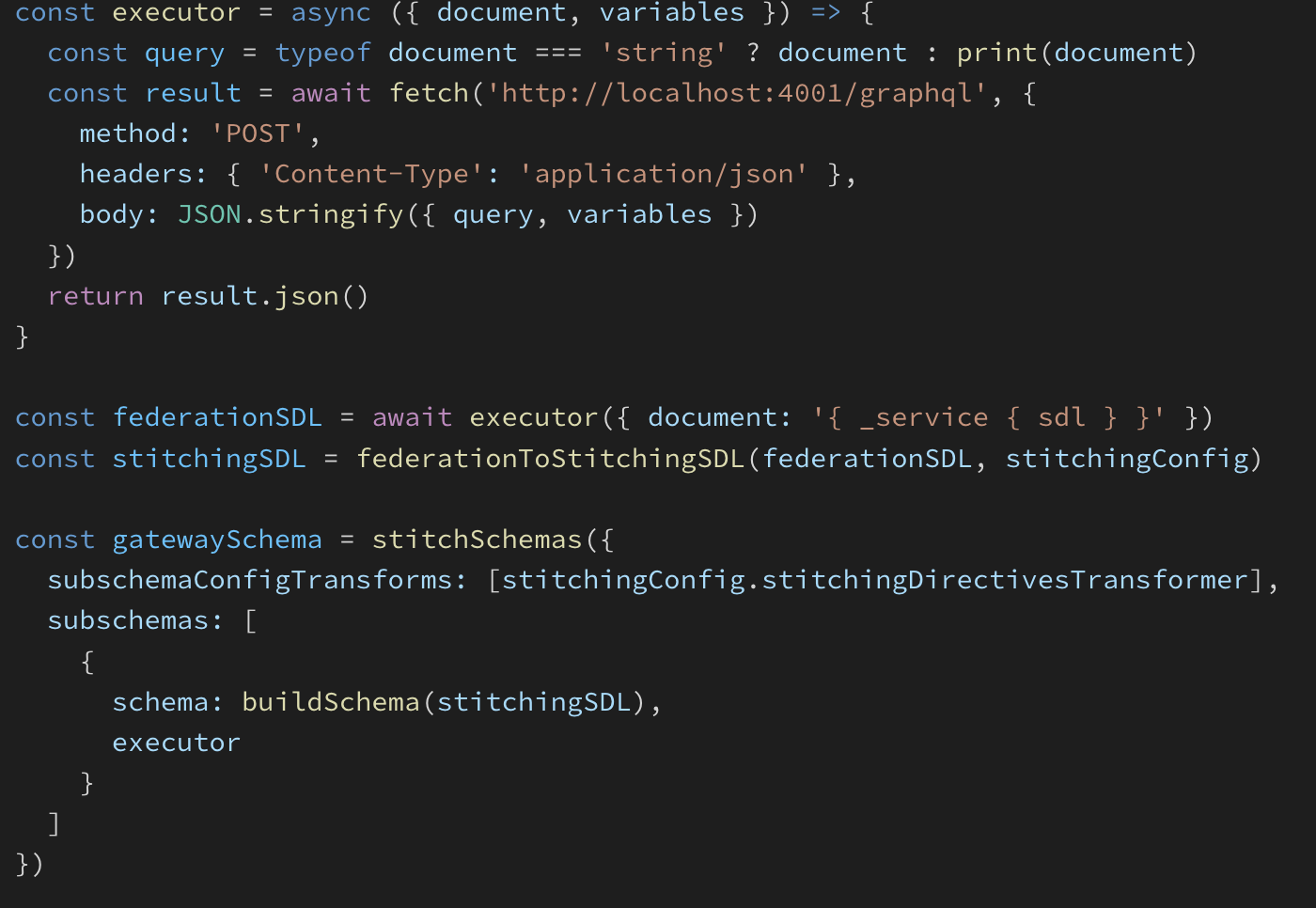
## Pitfalls
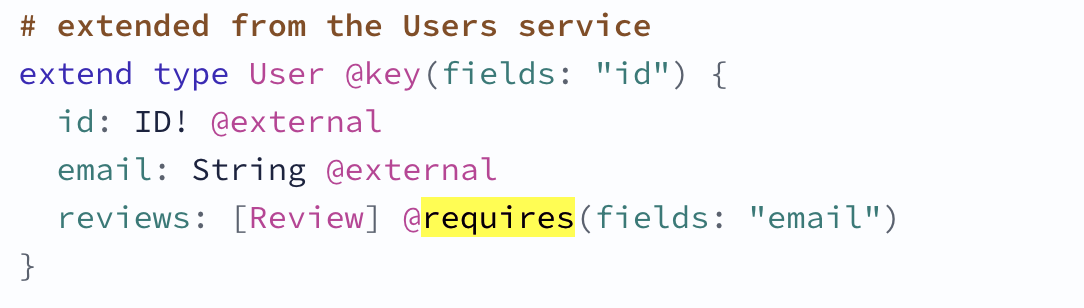
- Think about the federation when designing queries and entities, you could easily trigger multiple subgraph requests
## Pitfalls
- Avoid breaking changes, with federation you could break the graph for everyone
- Be careful about enums, interfaces, inputs with the same names (we have some things in place to prevent this)